[Tool] SVF Static Analysis
Static Value-Flow Analysis Framework
A scalable, precise and on-demand interprocedural program dependence analysis framework for both sequential and multithreaded programs.
Value-Flow Analysis
- resolves both control and data dependence.
- Does the information generated at program point A flow to another program point B along some execution paths?
- Can function F be called either directly or indirectly from some other function F 0?
- Is there an unsafe memory access that may trigger a bug or security risk?
Key features of SVF
- Sparse: compute and maintain the data-flow facts where necessary
- Selective : support mixed analyses for precision and efficiency trade-offs
- On-demand : reason about program parts based on user queries.
Client applications
- Static bug detection (e.g., memory leaks, null dereferences, use-after-frees and data-races)
- Accelerate dynamic analysis (e.g., Google’s Sanitizers and AFL fuzzing)
Several terms in static analysis
- Flow-sensitive
- Context-sensitive
- Heap-sensitive
- Field-sensitive

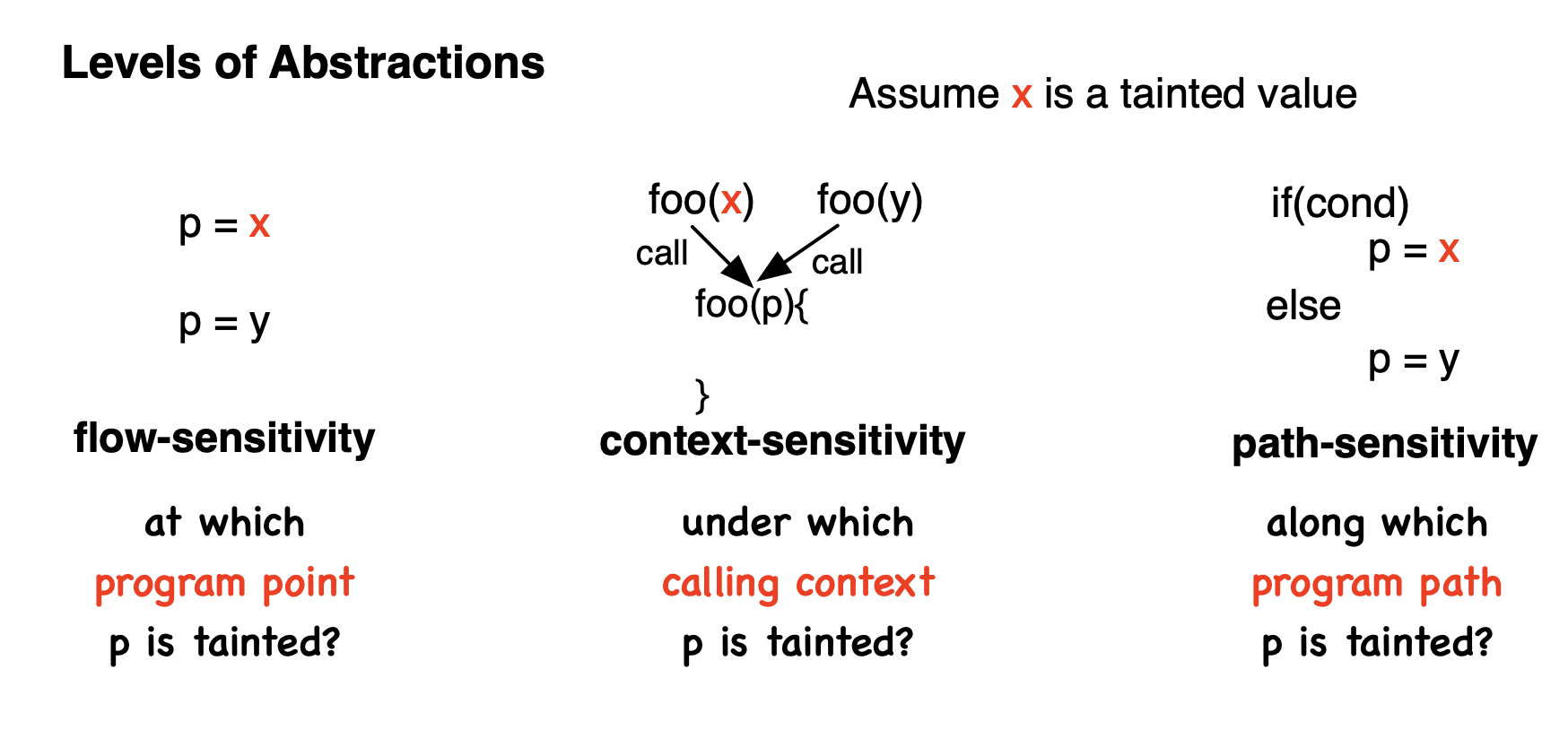
Graph Representation of Code
Representing a program’s control-flow (i.e., execution order) and/or data-flow (variable definition and use relations) using nodes and edges of a graph.
- Call graph: Program calling relations between methods
- Control flow graph: Program execution order between two
instructions.
- Intra-procedural control-flow graph: control-flow graph within a program method.
- Inter-procedural control-flow graph: control-flow graph across program methods. (ICFG)
- Constraint graph
- Program assignment graph
Pointer-to Analysis
Andersen’s analysis, a flow-insensitive, context-insensitive and path-insensitive Andersen’s analysis through analyzing the Constraint Graph of a program

Tool Usage
https://github.com/obfuscator-llvm/obfuscator/wiki

SVF
Install
1 | git clone https://github.com/SVF-tools/SVF.git |
Usage
Infer
Install
1 | VERSION=1.0.0; \ |
Usage
Here is a simple Java example to illustrate Infer at work.
1 | // Hello.java |
To run Infer, type the following in your terminal from the same
directory as Hello.java
.
1 | infer run -- javac Hello.java |
You should see the following error reported by Infer.
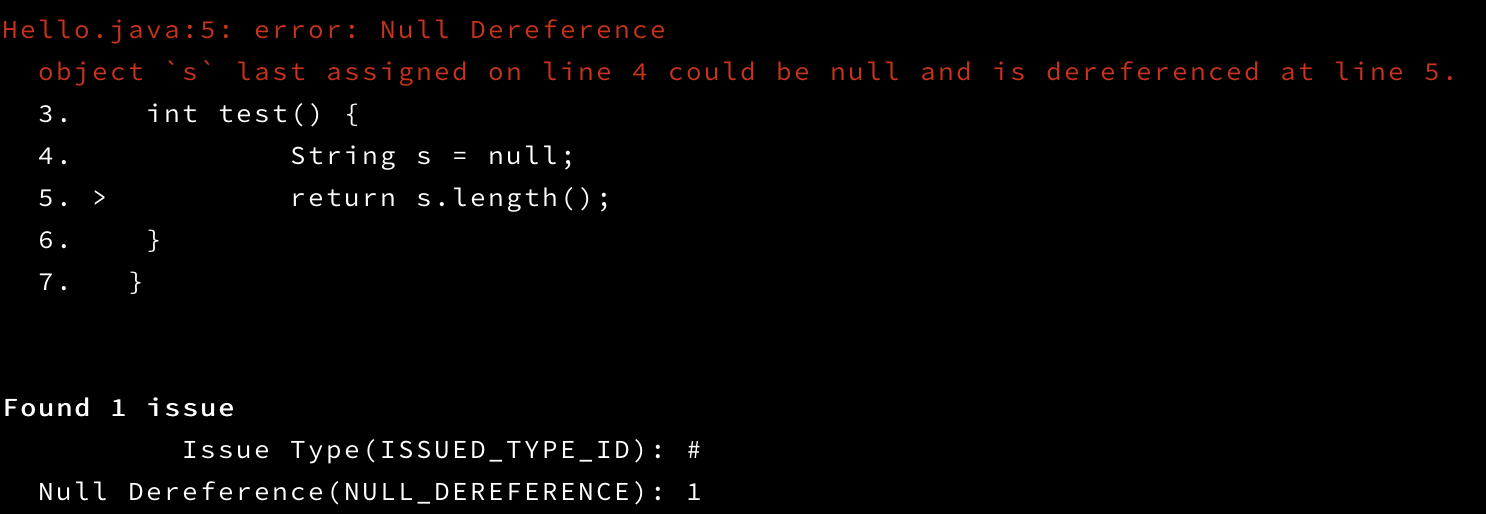
Here is a simple C example to illustrate Infer at work.
1 | // hello.c |
To run Infer, type the following in your terminal from the same
directory as hello.c
.
1 | infer run -- gcc -c hello.c |
You should see the following error reported by Infer.
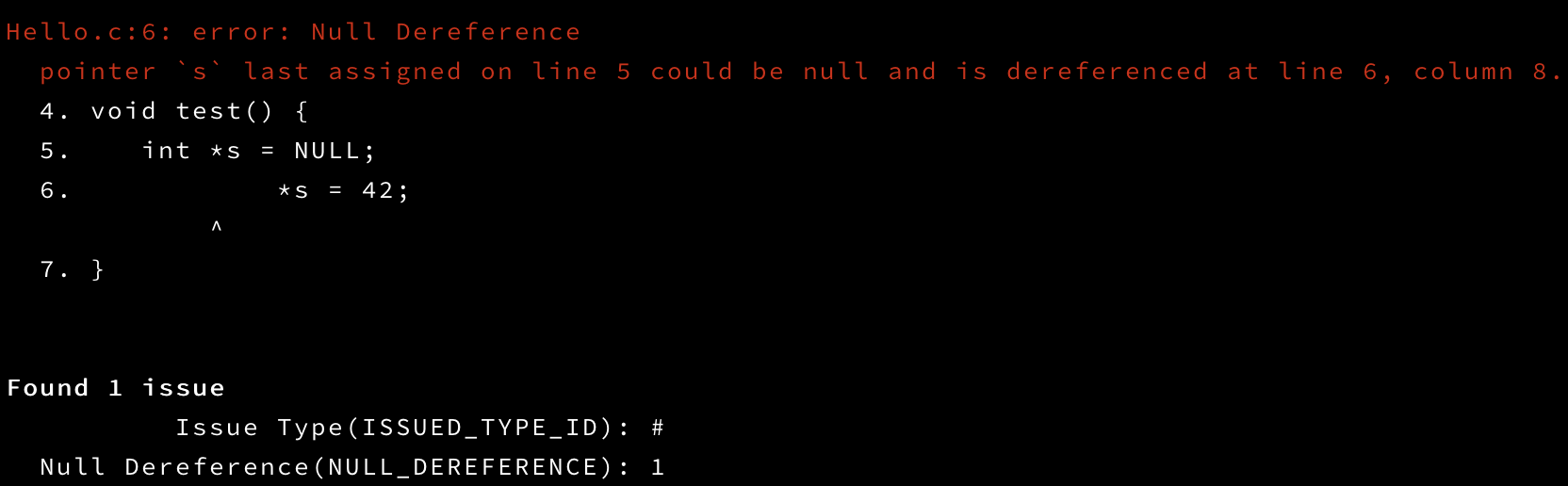
Clang Static Analyzer
1 |
|
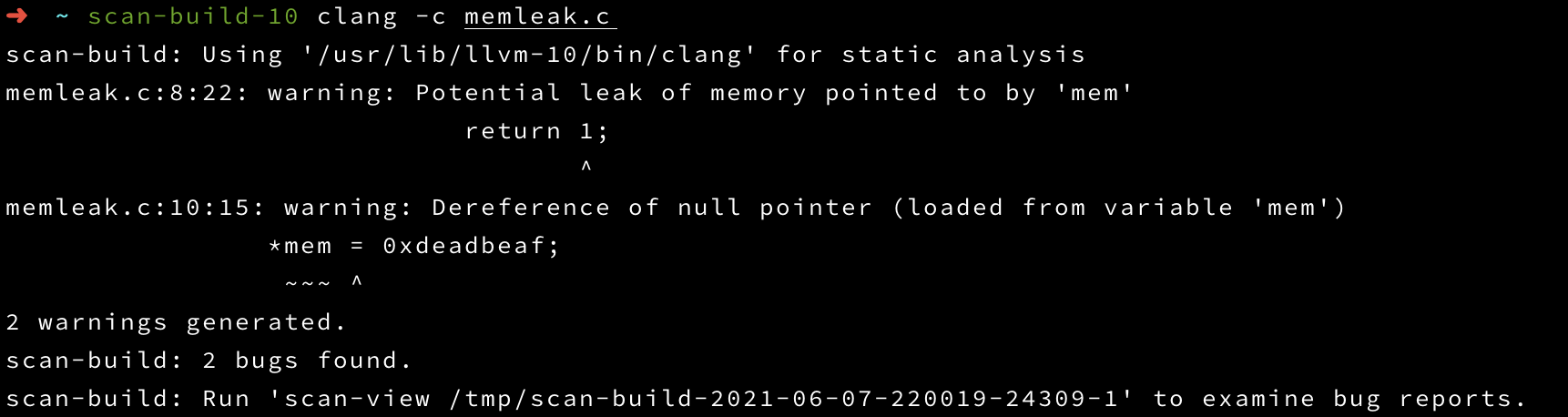
1 | <tr> |
1 | <tr> |