Gdb Usage
I would like to debug the following C program using gdb to check how
to set the memory content to run this program statically using BinCAT.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
int get_sign(int x){
if (x == 0){
return 0;
}
if (x < 0){
return -1;
}
return 1;
}
int main(int argc, char **argv){
int a = atoi(argv[1]);
int s = get_sign(a);
return 0;
}
Basically, I'm trying to set up argc and argv in correct memory location. The machine code of below C program is shown as below.
First, we compile this code with clang
and set the args
as 1.
1 | $ clang test.c -o test |
Second, we use layout asm
command to show the
disassembly of test and use b main
to set break at the
start address of main.
Third, we run the program using command r
, the program
stops at the instruction in address 0x400534
.
We can use p to show the value of register.
1 | (gdb) p $rsp |
ni
is used to execute instruction one by one. We stop at
0x400546
and check the value of edi
and
rsi
.
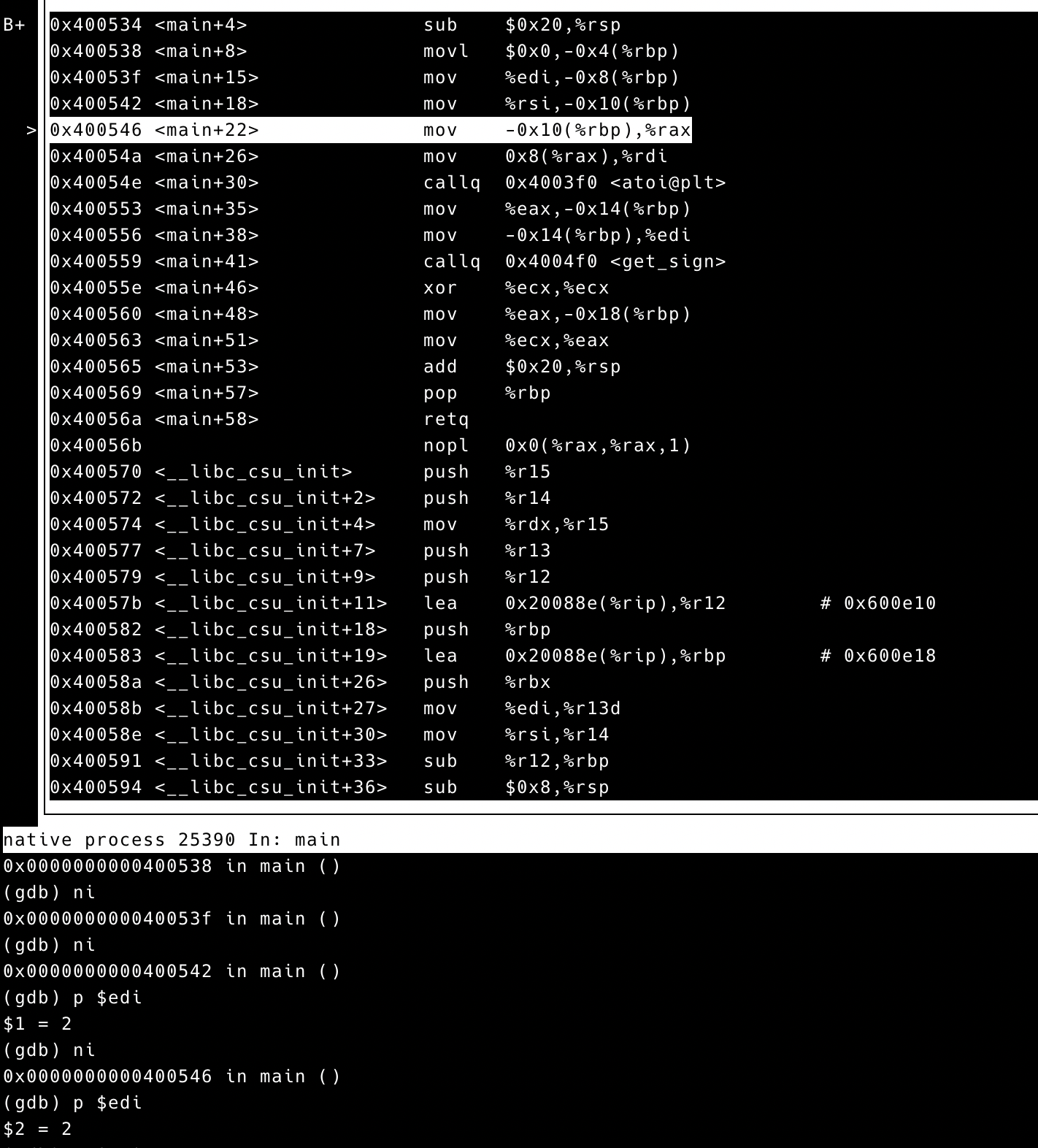
The result shows that edi=2
, which is exactly
argc
, cause there are two parameters.
rsi=0x0x7fffffffe308
which should be the value of
argv
. We check the memory content at address
$rsi
, then we can find that
argv[0]=0x7fffffffe581
and
argv[1] = 0x00007fffffffe594
. Further, we check the memory
content of argv[0]
and argv[1]
, the two
parameters are listed.
1 | (gdb) p /x $rsi |
Therefore, mov rax, [rbp+var_10]
will move the value of
argv to register rax
, then mov rdi, [rax+8]
will move the value of argv[1] to rdi
, which is the
paramter of atoi function.
1 | (gdb) p/x $rdi |
Based on the observation, we configure BinCAT to perform taint analysis.
1 | reg[rsi] = 0xb8001008 # argv |
The same as concrete execution, rsi
is argv,
argv[1]=0x300200
, and the input 1 is store at
argv[1]
.
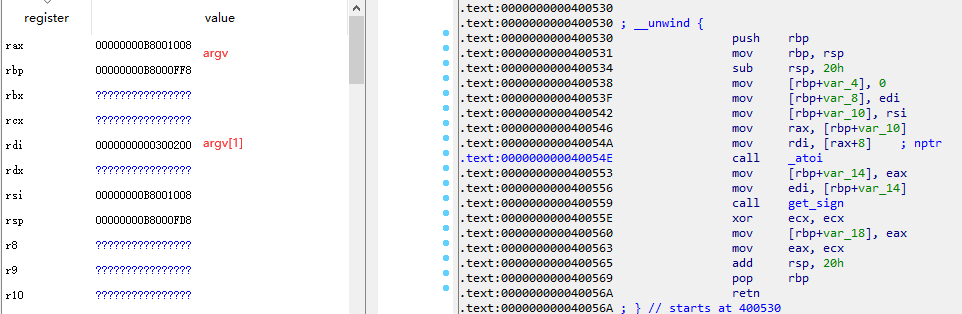